Day 9: Welcome to the world of Python OOP and Algo Trading
Welcome to Day 9 of 100 Days of Hell with Python Algo Trading. It’s not an easy journey—waking up at 2 am every day to record a video and then leaving for office at 7 am. But the lesson lies in the struggle. Today we will talk about a very important and powerful technique of Python—Object-Oriented Programming (OOP).
Object-Oriented Programming in Python: What and Why?
OOP is a concept that is essential to building any large, production-ready software.
Why is OOP important?
- Makes the code organized, readable and scalable
- Makes it easier to manage large projects
- Makes it modular by dividing each component into different blocks (objects)
OOP Concepts: Simple and practical examples
There are 6 main concepts of OOP, which are used in any Python project:
1. What is a Class?
- Class is a blueprint that tells how the object will be
- For example “Human” is a class which has some attributes (like two hands, two legs) and functions (like walking, thinking)
2. What is an Object?
- Object is a real-world instance of a class
- If “Human” is a class, then “Amit” and “Sita” can be two objects
3. Encapsulation
- Bundling data and functions in one place
- It maintains security and data integrity
4. Inheritance
- A class can inherit the properties of another class
- Like “Employee” class can take properties from “Human” class
5. Polymorphism
- A single function can behave in many ways
- Example: walk() function A child can walk in a different way and an old person can walk in a different way
6. Abstraction
- Showing important things and hiding other details
- Like when you drive a car, you don’t see the internal process of the engine, you just use gear, brake, steering
Importance of OOP in Algo Trading
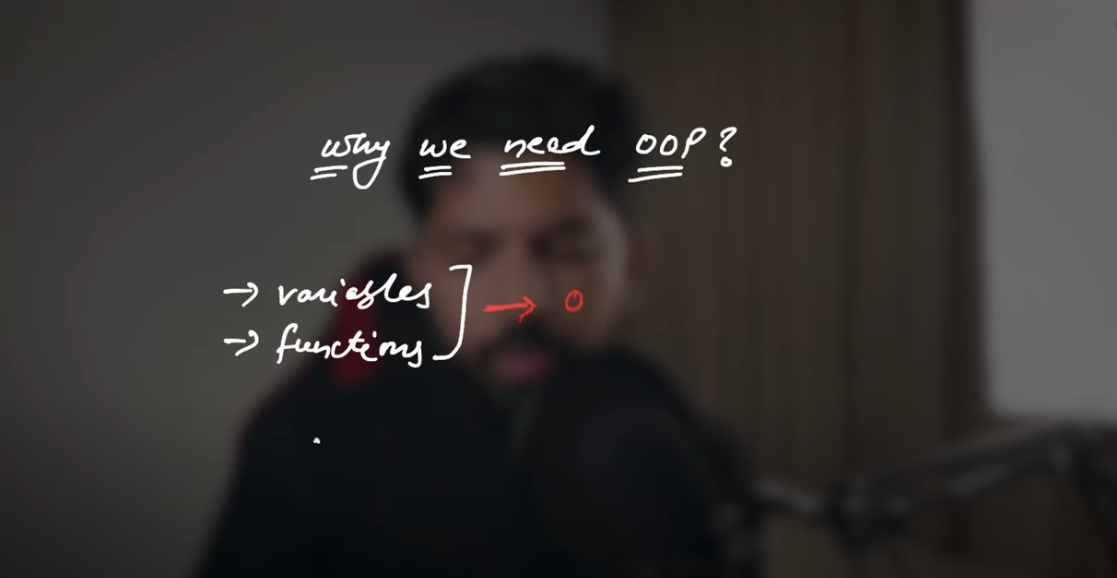
Object-Oriented in Algorithmic Trading with Python Programming is helpful at many levels:
- Structuring Quantitative Trading Strategies
- Defining strategies into separate classes
- Indicators, signals, execution—all as separate objects
- Uses in Crypto Trading Automation
- Easy to convert every exchange, API, and strategy into an object
- Makes code reusable, maintainable, and secure
- Role of OOP in Best Algorithmic Trading Software in USA
Platforms like Freqtrade, Zipline, QuantConnect operate on OOP-based architecture
Strategies are deployed using classes and modules
OOP in Quantitative Analysis for Trading in Singapore
Managing Trading Bots, Indicators, and Signal Analysis by creating separate objects
Making strategy building and risk management more modular
A simple example: Human Class
Suppose we have a Human class:
class Human:
def __init__(self, name):
self.name = name
self.legs = 2
self.hands = 2
def walk(self):
print(f”{self.name} is walking”)
def think(self):
print(f”{self.name} is thinking”)
Now we can create an object of it:
person = Human(“Aakash”)
person.walk()
This example shows how real-life entities can be inserted into Python’s OOP structure.
Watch this Day 9 video tutorial
Day 9: OOP Part1