Algorithmic Trading with Python: Day 11 – Understand the real meaning of Encapsulation
Hello friends!
Welcome to Day 11 of 100 Days of Hell with Python Algo Trading. Today we will talk about Encapsulation – which is a very important but most misunderstood concept of Object Oriented Programming (OOP).
By now you must have read about it in books – “Encapsulation means binding data (attributes) and methods working on that data in a single class, so that accidental interference and misuse can be avoided.”
But have you ever wondered why we need it?
Today we will understand its real meaning and importance with an easy example.
Why is Encapsulation important?
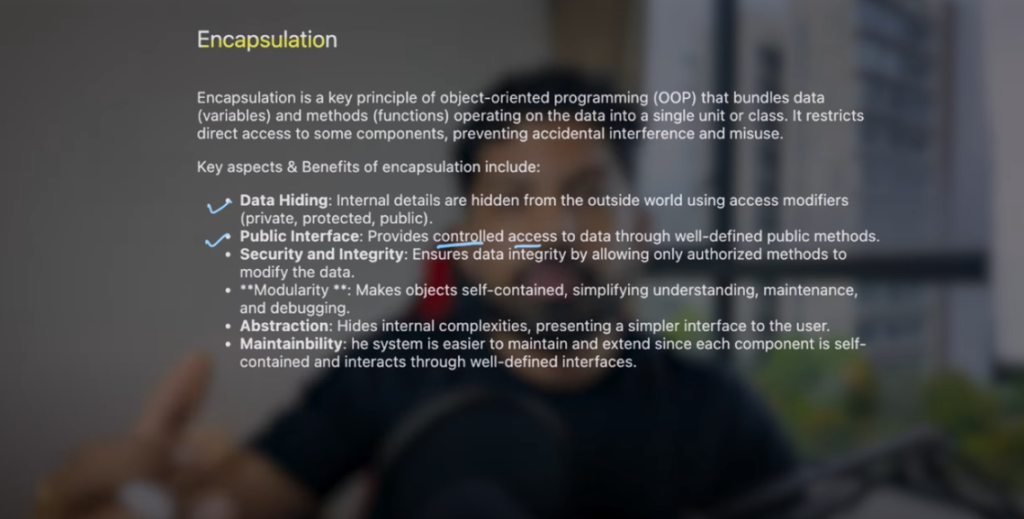
1. Data Hiding
Encapsulation allows us to hide data. That is, no user can directly access or modify the data inside our object. For this we use access modifiers – such as private, protected, and public.
2. Public Interface
We can create a clean and controlled interface of our object so that only necessary and secure data is allowed to be accessed.
3. Modularity
Each class is like a separate unit, which makes our code more readable, maintainable and easy to debug.
4. Abstraction
The user does not care about the internal complexity of the system. With the help of encapsulation, the user gets only the functionality that is necessary.
5. Maintainability
If we have to change something in the future, then we can easily modify the encapsulated components individually.
Let's understand with an example:
Structure of a simple Trading Bot
class AutoTradingBot:
def __init__(self, threshold):
self.__threshold = threshold # threshold value (private)
self.__position = None # current position
self.__price_data = [] # market price data (empty list)
def fetch_market_data(self):
pass # fetch data from here
def fetch_latest_price(self):
pass # update latest price
def evaluate_price(self):
pass # compare with threshold
def execute_trade(self):
pass # write buy/sell logic
def get_threshold(self):
return self.__threshold # Encapsulated data access
def set_threshold(self, value):
self.__threshold = value # Controlled modification
Note: We made __threshold, __position and __price_data private And we control them through public methods.
Also understand Instance Variables:
class TradingBot:
def __init__(self, name, initial_cash):
self.name = name
self.cash = initial_cash
bot1 = TradingBot(“Apple”, 1000)
bot2 = TradingBot(“Microsoft”, 2000)
print(bot1.name) # Output: Apple
print(bot2.cash) # Output: 2000
Each object (bot1, bot2) has its own instance variables — that is, multiple objects can be created from the class and each object has its own data.
Benefits at a glance:
Benefits
Data Hiding
Public Interface
Modularity
Abstraction
Maintainability
Meaning
Hiding sensitive data from the outside world
Accessing data securely
Breaking code into pieces – easy to understand and change
Giving only the necessary functionality to the user
Easy to change in the future
Encapsulation is not just a definition — it is a concept about how we can make our code secure, organized, and easy to maintain.
In today’s lesson, we learned how to create a better Trading Bot in Python through encapsulation.
Watch this Day 11 video tutorial
Day 11: Object-Oriented Programming Part – 3