Matplotlib is a powerful Python library that is widely used for data visualization. Creating graphs and charts is essential for effectively analyzing data in algorithmic trading and crypto trading. In this guide, we will discuss the usage of Matplotlib and its various plots that are useful for quantitative traders.
What is Matplotlib?
Matplotlib is an open-source Python library that helps create high-quality graphs and charts. It is popular among data scientists and developers because it is simple and easy to use.
Why use Matplotlib?
- Simplicity: The syntax of Matplotlib is straightforward and easy to understand, allowing even new users to learn it quickly.
- Versatility: It provides the ability to create different types of plots such as line plots, scatter plots, bar charts, histograms, pie charts, etc.
- Customization: Users can customize the graph colors, labels, titles, etc. as per their requirements.
How to use Matplotlib?
Installation: First, install Matplotlib:
pip install matplotlib
Import: After installation, import it into your Python script:
import matplotlib.pyplot as plt
Prepare data: Prepare the data you want to visualize.
Create plot: Create the graph using the appropriate plot function.
Customize: Add graph titles, labels, etc.
Display: Finally, display the graph:
plt.show()
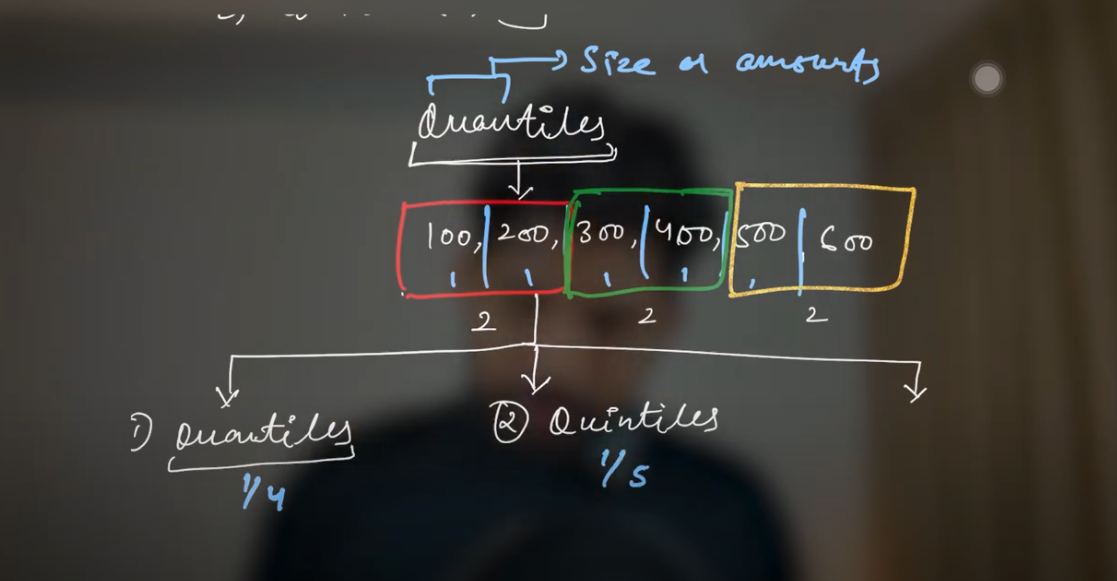
Different types of plots
1. Line Plot
Line plots are used to show the trend of data over time.
import matplotlib.pyplot as plt
# Data
years = [2018, 2019, 2020, 2021, 2022]
prices = [100, 150, 200, 250, 300]
# Create a line plot
plt.plot(years, prices)
plt.title(‘Trend of prices over years’)
plt.xlabel(‘Year’)
plt.ylabel(‘Price’)
plt.show()
2. Scatter Plot
A scatter plot is used to display the relationship between two variables.
import matplotlib.pyplot as plt
# Data
investment = [1000, 2000, 3000, 4000, 5000]
returns = [50, 80, 90, 100, 150]
# Create a scatter plot
plt.scatter(investment, returns)
plt.title(‘Relation between investment and returns’)
plt.xlabel(‘Investment Amount’)
plt.ylabel(‘Returns’)
plt.show()
3. Bar Chart
Bar charts are used to compare different categories.
import matplotlib.pyplot as plt
# Data
assets = [‘stocks’, ‘bonds’, ‘real estate’, ‘cash’]
allocation = [50, 20, 20, 10]
# Create a bar chart
plt.bar(assets, allocation)
plt.title(‘Portfolio Allocation’)
plt.xlabel(‘Assets’)
plt.ylabel(‘Percentage’)
plt.show()
4. Histogram
Histogram is used to visualize the distribution of data.
import matplotlib.pyplot as plt
import numpy as np
# Data
returns = np.random.normal(0.1, 0.02, 1000)
# Create a histogram
plt.hist(returns, bins=20, edgecolor=’black’)
plt.title(‘Distribution of Returns’)
plt.xlabel(‘Returns’)
plt.ylabel(‘Frequency’)
plt.show()
5. Pie Chart
Pie charts are used to show the percentage of different parts of a population.
import matplotlib.pyplot as plt
# Data
assets = [‘stocks’, ‘bonds’, ‘real estate’, ‘cash’]
allocation = [50, 20, 20, 10]
# Create a pie chart
plt.pie(allocation, labels=assets, autopct=’%1.1f%%’, startangle=140)
plt.title(‘Portfolio Allocation’)
plt.show()
Using matplotlib, data can be effectively analyzed in algorithmic trading and crypto trading. It is an essential tool for quantitative traders,
Watch this Day 33 video tutorial
Day 33: Matplotlib In Python