Basics of Algorithmic Trading with Python
Python has gained immense popularity in the field of algorithmic trading due to its simplicity, extensive libraries, and strong community support. Whether you are a beginner or an experienced trader, learning Python can significantly enhance your ability to develop trading strategies, analyze market data, and execute trades automatically.
In this guide, we will cover the fundamental concepts of Python and how it can be applied to algorithmic trading.
So we will be learning algo trading for the next 100 days so you will become an expert algo Trader after 100 days so first thing first we have to Learn Python okay so what is the definition of python so python is a high-level interpreted programming language known for its clear syntax what is the meaning of interpreted basically there are two concepts one is compiler and second is interpreter so compiler generally compiles the whole program in one go and it executes this and in interpreter what it does it execute the program line byline so we can say python is an interpreted programming language and also it’s known for its clear syntax the code is very beautiful and it supports multiple programming paradigms.
means you can code in procedural object-oriented and functional programming so we will be learning object-oriented programming shortly after a few videos and also with the help of python we can do multiple things like it can be used for web development.
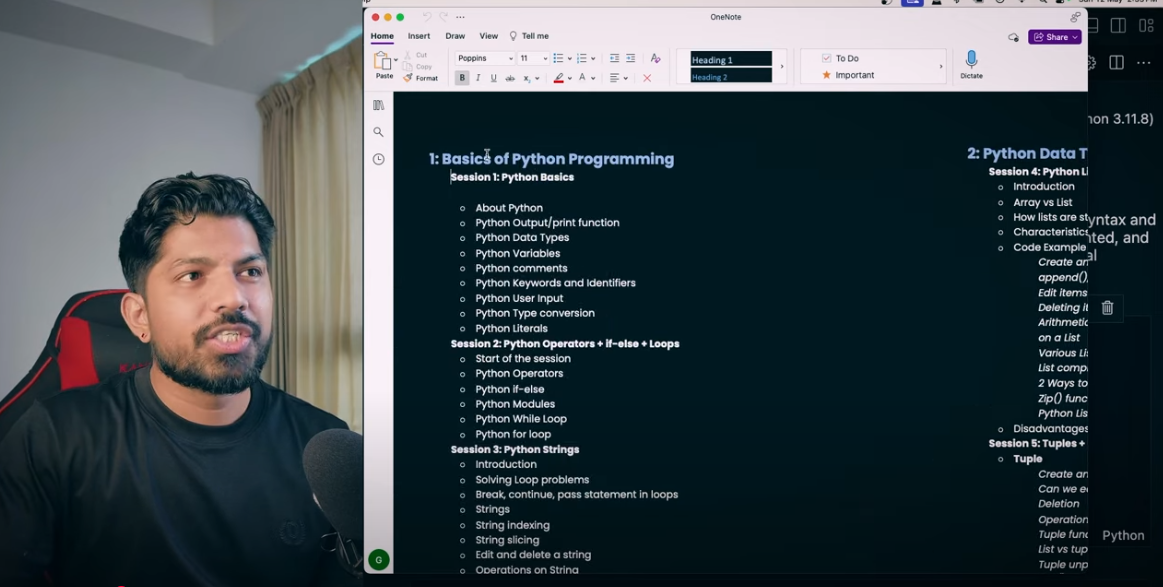
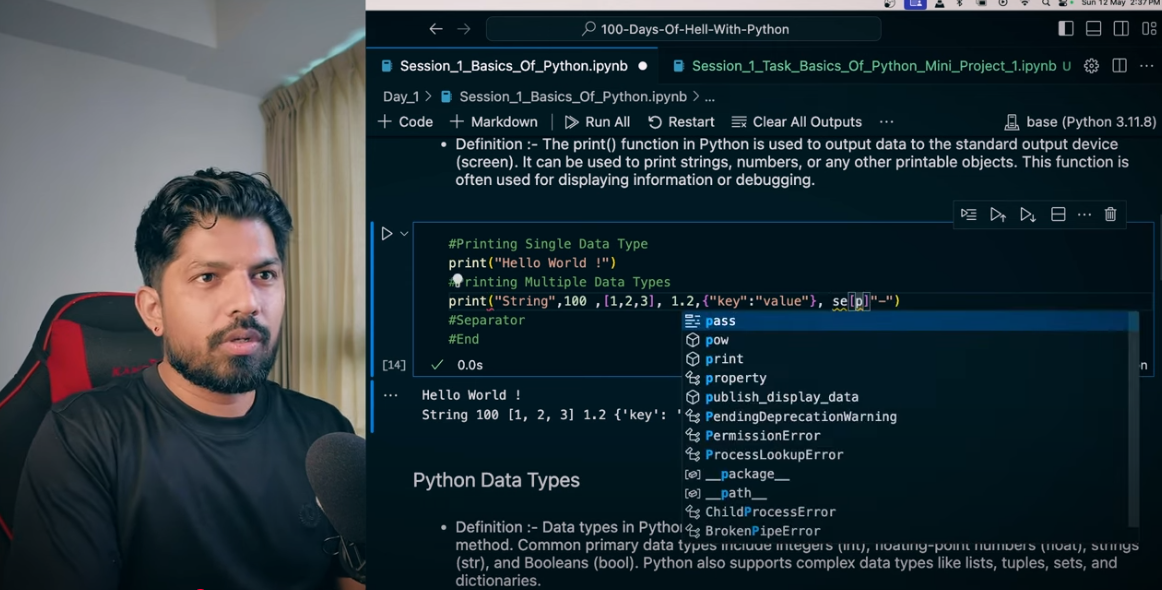
It can be used for data analysis and also you know that we can use use this in artificial intelligence scientific computing and much more so one question arises why python because of its design philosophy as I have already explained the synex of python is very clear and because of its readability correct and second is batteries included means it has so many functions which makes our life so easy for example to reverse a string it has a single function but in other languages like C C++ Java we have to write a longer code.
Right so that’s why there are so many functions which you can use straight away and it reduces your lines of code and third is general purpose programming language as I already explained we can use it for web development artificial intelligence data analysis and so many things and almost anything and everything we can use Python and the fourth is libraries and Community because it has so many libraries so you can straight away use those pre-coded libraries and you can make your any application way faster than any other language.
Also it has a huge community so whenever you are stuck in any condition you can always ask for help and also you can contribute from your side also so why python for algo trading the first is easy to Learn Python is very easy and anyone can learn in just one or two weeks and they can start coding and second is proximity with maths because it has so many pre-built modules and libraries which you can directly use whenever you want to perform any mathematical operations so it makes your life.
So easier and also the community is very huge and there are so many people who are are always eager to help you so that’s the basic reason behind that so let’s move to the next section but before that and let me show you the process we are going to follow okay so this is how we going to follow the process so we will be having 100 sessions and in which we’re going to cover each and everything right I’ll post this link in the description you can go and check that how we are going to cover the topics so first we will learn about the basics of Python Programming which will’ll be having few sessions you can go and check out then.
We will learn about the python data types then the next is objectoriented programming then Advanced python then the the next will be the libraries of python which is numpy and pandas which is very important you will be using virtually everywhere right and then next will be Advanced pandas then the next will be the data visualization and and the next will be the data analysis right so this is the plan for the next 30 days and remaining 70 days I will be updating in the future videos.
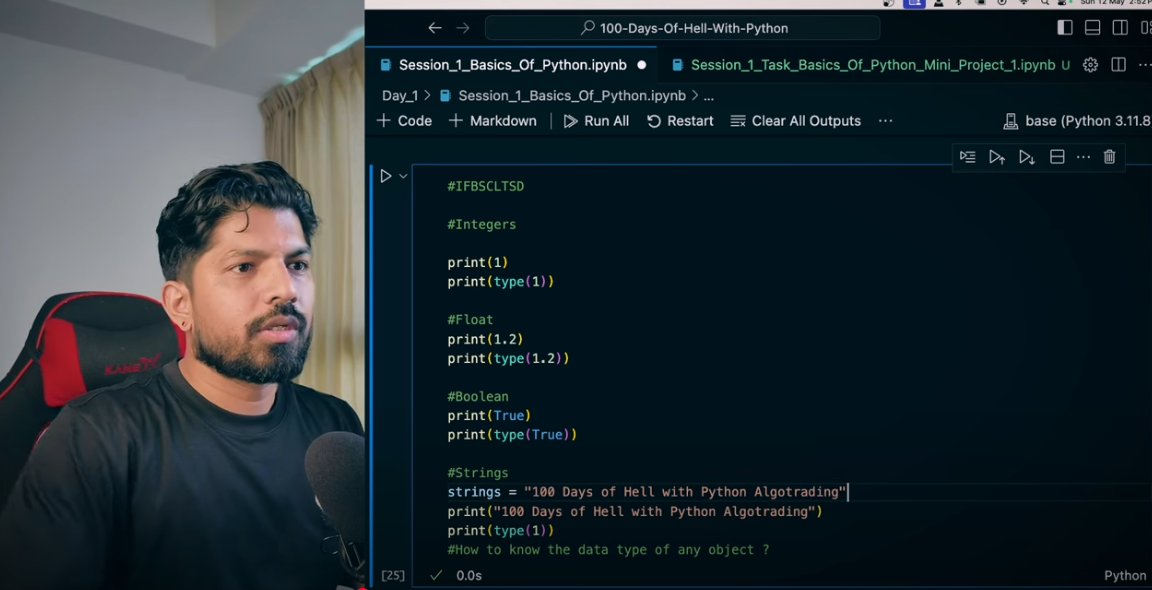
Watch this Day 1 video tutorial
Best Python Libraries for Algo Trading:
Pandas & NumPy – Data analysis & handling
TA-Lib – Technical indicators for quantitative analysis in Singapore
Freqtrade – Open-source crypto trading bot
Setting Up Your Algorithmic Trading Environment
Step 1: Install Python & Required Libraries
pip install pandas numpy ta freqtrade
Step 2: Set Up a Trading API (Binance, Coinbase, etc.)
Step 3: Implement a Basic Trading Strategy
import pandas as pd
data = pd.DataFrame({‘Price’: [45000, 46000, 47000], ‘Moving_Avg’: [45500, 45700, 46500]})
data[‘Signal’] = data[‘Price’] > data[‘Moving_Avg’]
print(data)
Introduction to Freqtrade Strategy for Crypto Trading
Freqtrade is one of the best algorithmic trading software in the USA for automating crypto trades.
How to Install Freqtrade?
git clone https://github.com/freqtrade/freqtrade.git
cd freqtrade
./setup.sh –install
How Freqtrade Helps?
Supports backtesting & paper trading
Implements custom crypto trading strategies
Ideal for quantitative traders in Singapore & the USA
Developing a Simple Trading Strategy
Using RSI Indicator for Trade Execution
import talib
# Sample price data
prices = [45000, 45500, 46000, 47000, 46500]
# Calculate RSI
rsi = talib.RSI(prices, timeperiod=14)
if rsi[-1] < 30:
print(“Buy Signal”)
elif rsi[-1] > 70:
print(“Sell Signal”)
6. Backtesting & Optimizing Your Trading Strategy
What is Backtesting?
Backtesting helps traders test their strategies using past data before deploying them live.
How to Backtest in Freqtrade?
freqtrade backtest –strategy MyStrategy
Go ahead—challenge yourself and solidify your learning!
Day 1: Python Basics