Welcome to Day 6: 100 Days of Hell with Python Algo Trading
Today we will take a closer look at two of the most important data types in Python—Sets and Dictionaries. Whether you are into Quantitative Trading or Crypto Strategies, mastering both of these concepts is a must. Today’s session started at 3:30 am in Singapore, and this is how we learn the true meaning of consistency.
If you are feeling demotivated, remember: success is another name for hard work and consistency.
What are Sets?
Sets are an unordered collection in which every element is unique and immutable.
However, the set itself is mutable, meaning you can add or remove new items from it.
Features of Sets:
- Unordered (no fixed order)
- No duplicates allowed
- Mutable in nature
Elements must be immutable (mutable elements like lists will not work)
Set Operations
1. Union
Combining two sets to create a new set containing all unique elements.
a = {‘Apple’, ‘Google’, ‘Tesla’}
b = {‘Tesla’, ‘Amazon’, ‘Microsoft’}
u = a.union(b)
# Output: {‘Apple’, ‘Google’, ‘Tesla’, ‘Amazon’, ‘Microsoft’}
Duplicate elements like ‘Tesla’ will appear only once.
2. Intersection
Shows the common elements present in both sets.
i = a.intersection(b)
# Output: {‘Tesla’}
3. Difference
Removing one set from another.
a – b:
a.difference(b)
# Output: {‘Apple’, ‘Google’}
b – a:
b.difference(a)
# Output: {‘Amazon’, ‘Microsoft’}
4. Symmetric Difference
The set of unique elements remaining after removing common elements from both sets.
a.symmetric_difference(b)
# Output: {‘Apple’, ‘Google’, ‘Amazon’, ‘Microsoft’}
Additional Set Properties
Disjoint Sets
If two sets do not have any common element, then they are called disjoint.
a = {‘Apple’, ‘Google’}
b = {‘Amazon’, ‘Microsoft’}
a.isdisjoint(b) # Output: True
But our main sets (a and b) had ‘Tesla’ in common, so:
a.isdisjoint(b) # Output: False
Subset and Superset
Subset:
If all elements of set A are also present in set B:
a = {‘Apple’, ‘Google’}
b = {‘Apple’, ‘Google’, ‘Amazon’}
a.issubset(b) # Output: True
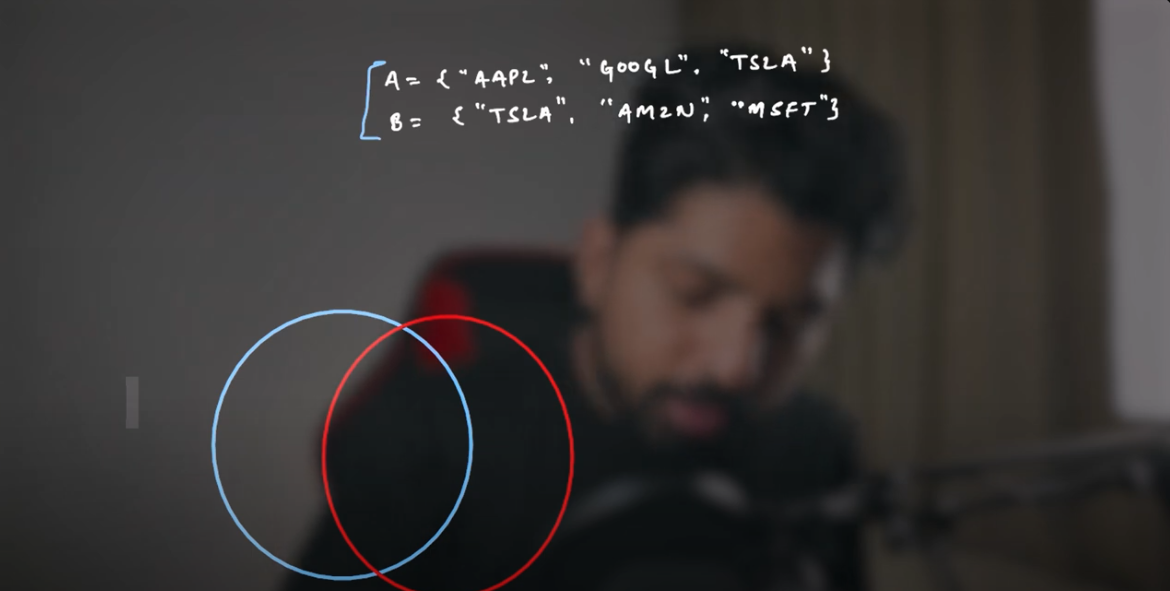
Superset:
If all elements of A are present in set B:
b.issuperset(a) # Output: True
Uniqueness Test
If a set has duplicate values, then it will not be called unique.
b = {‘Apple’, ‘Apple’, ‘Google’}
len(b) # Output: 2, because duplicate is removed
Quick Set Examples for Practice
# Set Creation
stocks_a = {‘Apple’, ‘Google’, ‘Tesla’}
stocks_b = {‘Tesla’, ‘Amazon’, ‘Microsoft’}
#Union
print(stocks_a | stocks_b)
#Intersection
print(stocks_a & stocks_b)
#difference
print(stocks_a – stocks_b)
print(stocks_b – stocks_a)
# Symmetric Difference
print(stocks_a^stocks_b)
Watch this Day 6 video tutorial
Day 6: Python Sets and Dictionaries