What is Algorithmic Trading?
Algorithmic trading is a modern technology that gives traders the ability to implement their trading decisions with accuracy and speed. Algorithmic trading with Python is revolutionizing today’s world, especially in the field of quantitative trading and crypto automation. This is a perfect starting point to understand the best algorithmic trading software in USA and quantitative analysis in Singapore.
Why choose Python for Algorithmic Trading?
The Python programming language is most commonly used in algorithmic trading due to its simplicity, readability, and large library support. With Python, you can perform tasks such as data analysis, backtesting, and automated strategies.
Freqtrade Strategy – Open Source Tool
Freqtrade is a powerful open-source algorithmic trading tool that works with Python. It helps to backtest and optimize automated trading strategies. Users can create their own strategies in it, making it a multipurpose tool.
Why do we need Python functions?
What is a function?
A function is a block of code that performs a certain task and can be used multiple times. For example, if you need to perform addition multiple times in a program, create a function named ‘add()’ once, and just call it each time.
Function definition:
def add(a, b):
return a + b
Here def is a keyword, add is the name of the function, (a, b) are parameters and return returns the result.
Make the code reusable and modular
The biggest strength of functions is that it prevents code repetition and gives a clean modular structure. For example, if you are making a calculator, you can create separate functions like add(), subtract(), multiply() and divide(). This makes the code readable, easy to debug and scalable.
How to define functions?
Follow these simple steps to create a function:
Start with the def keyword
Name the function (e.g.: calculate_sum)
Give parameters in parentheses (e.g.: a, b)
: Put a colon
Write the code in an indented block
def calculate_sum(a, b):
result = a + b
return result
Now this function can be called repeatedly with inputs:
print(calculate_sum(5, 7)) # Output: 12
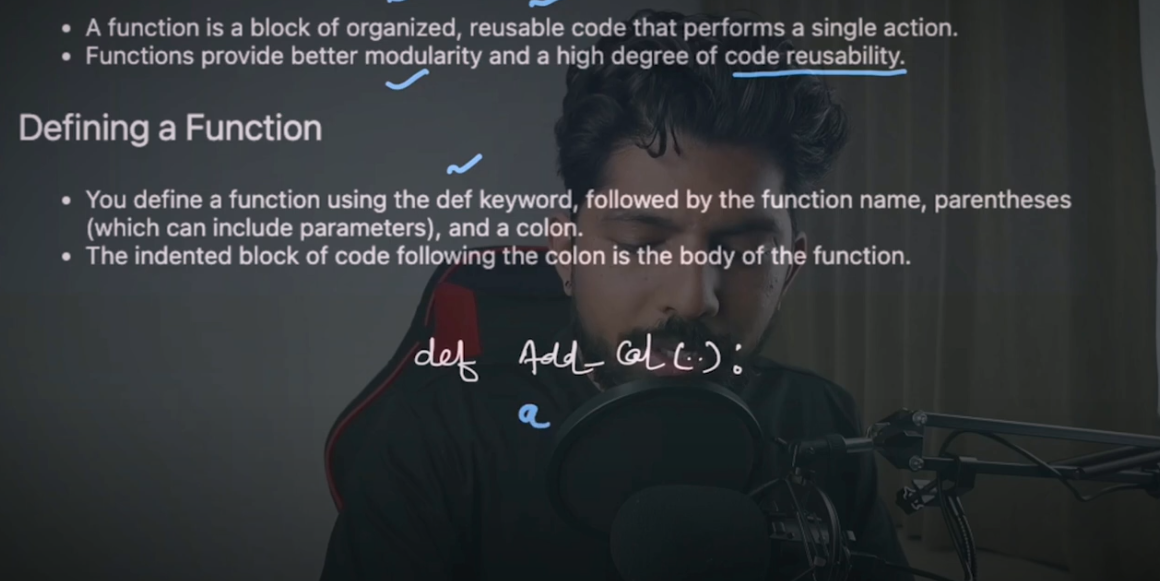
Where are functions used?
- Quantitative trading: data analysis, indicator calculations, trading strategies
- Crypto trading automation: signal generation, order execution
- Backtesting and risk analysis: testing function-based strategies on historical data
- UI/UX interface: using backend functions in web applications
Why are functions important in algorithmic trading?
Algorithmic trading requires the same logic to be repeated over and over again – like calculating indicators, generating buy-sell signals, risk control, etc. Functions are the best solution for all these tasks as they make the code DRY (Don’t Repeat Yourself).
Use of Algorithmic Trading in USA and Singapore
In USA, traders use cutting-edge softwares that are based on Python and functions.
In markets like Singapore, quantitative finance is growing rapidly, and functions make systematic trading modular and scalable.
Watch this Day 7 video tutorial
Day 7: Python Functions
13/20