Python If-Else & Loops in Algorithmic Trading & Crypto Strategies
Algorithmic trading is transforming the financial markets, enabling traders to automate strategies for maximum efficiency. In this video, we explore algorithmic trading with Python, perfect for quantitative traders looking to enhance their strategies. You’ll discover top crypto trading strategies, the best algorithmic trading software in the USA, and how quantitative analysis for trading in Singapore is evolving. We also dive into Freqtrade strategy, a popular choice for automated trading. Let’s get started!
So before starting this session I would like to clarify some queries raised by some subscribers if you have any query you can connect with us on any social media platform mentioned in the description and so many people even ask me where you can find the mcqs multiple choice questions after the session so those questions can help in your learning or retention so for that also you can find the link in the description and you can just attempt those questions those are very useful well researched.
Well curated questions specifically for that particular day session then you can find the session jupyter notebook and the task sheet which involves various good questions and have a mini project so both the sheets of session notebook and the task I have committed to the repository so you can find from there I’ll mention the link in the description now let’s quickly move to the today’s session that is python operators and conditional statements which is if else we’ll be learning those topics which are very crucial because if your fundamentals are strong.
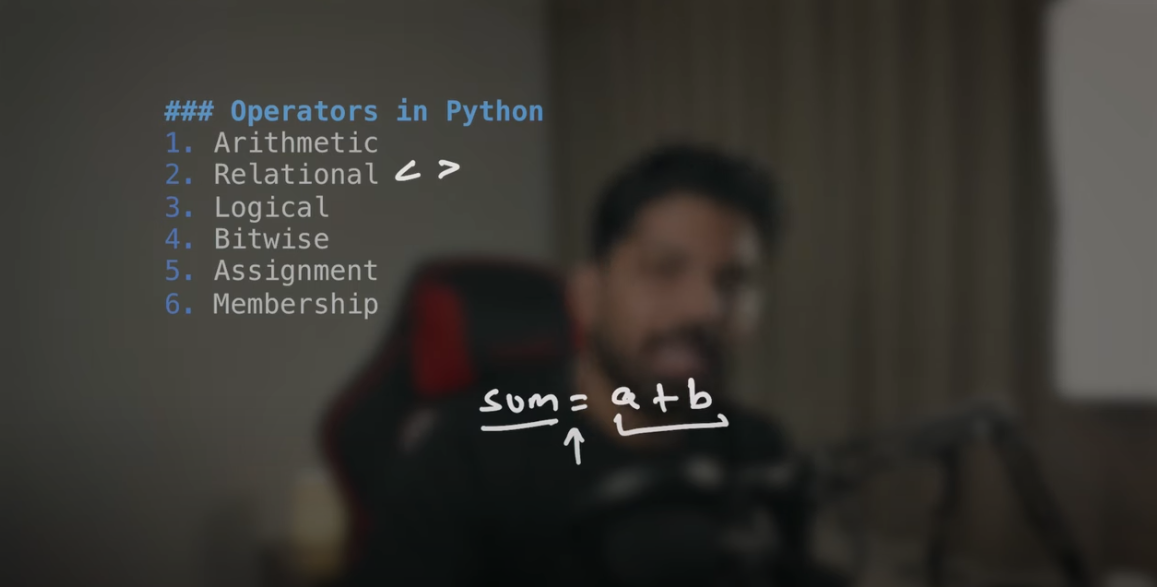
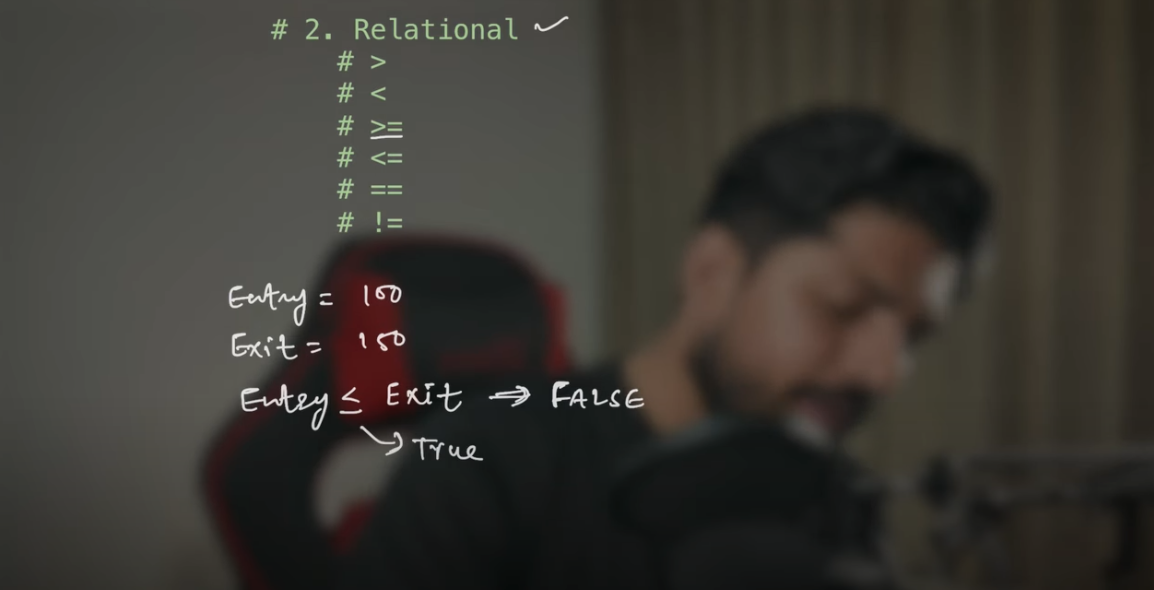
Then definitely those will help you a lot in the future and also you know that when you try to work on any big problem and then you find that you are not even able to resolve those fundamental queries those fundamental questions which are very basic and because of that I giving so much of emphasis on these fundamentals and once we are done with this and then you will see that you are much more confident in handling those big task okay so let’s quickly move to the today’s topic that is python operators and conditional statements which is if else and if L if okay so so first let me explain you okay and then.
We’ll jump on the uh coding examples so let’s understand what are the operators so operators are the special symbols or you can say keywords which are used to perform some operations on variables and values right so you can say the symbol between variable and value so for example so like we say uh sum equals to A plus b right here sum is the variable and a plus b is the value of that variable correct and here this symbol is known as The Operators and python supports various kinds of operators for example we have arithmetic in which we perform addition subtraction division multiplication then we have relational in that we calculate the less than greater than correct and then next we have logical and in logical.
We have the and or not then we have bitwise so bitwise operators are used to per perform bit level operations which also have and or exor and left shift right shift we will shortly understand all those then we have assignment operator which is the equal to sign and last but not the least we have membership operators which is in and not in okay so let’s try to understand them one by one okay so the first type of operators are the arithmetic operators correct in this we have addition which is plus then subtract fraction which is this sign and then we have multiplication uh division integer division then modulo and then power off so how does this work for example.
We have a = to 5 and b equal to 2 correct so if you try to perform addition on this then the results will be 5 + 2 which is 7 which is basic maths then when we perform subtraction which is 5 – 2 we will get three then we perform multiplication which is 5 multiplied by 2 we will get 10 then we have division we will get here 2.5 correct a float value then we have the integer division which is very useful and very important right so here what we will get when you perform five integer division two then we will get only two because it will ignore the 0.5 which is not an integer correct so we will only get the integer value sometimes it’s very useful I’ll just show in the example then we have the modulo so in modulo we get one we only get the remainder of that particular operation.
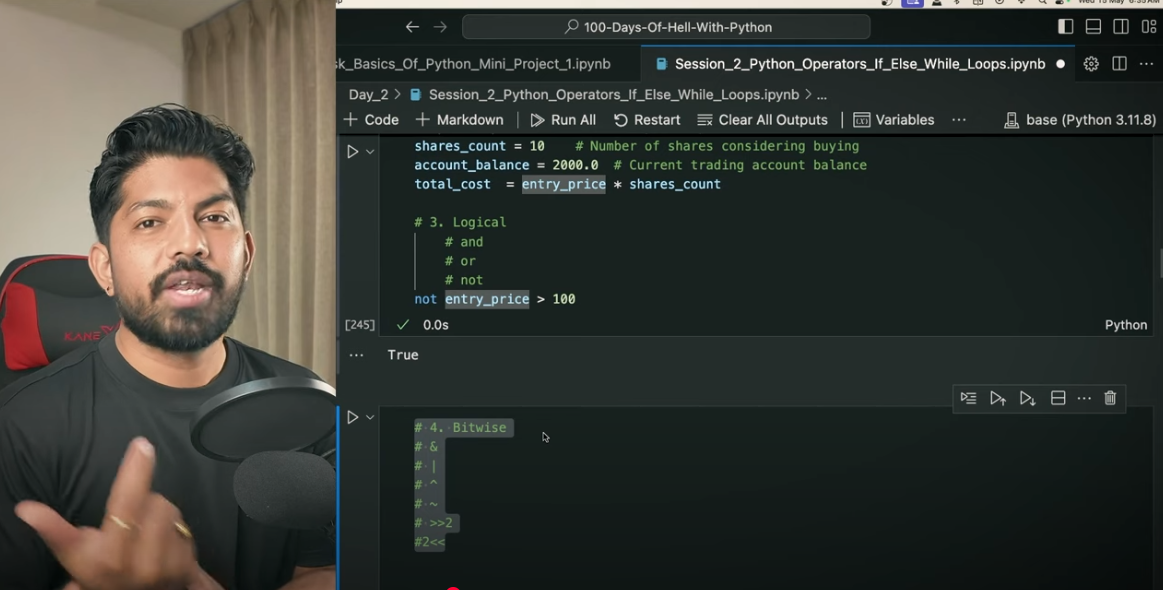
Watch this Day 2 video tutorial
Example: Basic If-Else for Trade Execution
# Sample trade data
price = 45000
moving_average = 46000
if price > moving_average:
print(“Sell BTC-USD”)
else:
print(“Buy BTC-USD”)
Using If-Elif-Else for Multi-Condition Trading
Why Use If-Elif-Else?
Handles multiple trade conditions
Improves quantitative analysis for trading in Singapore
Example: Multi-Condition Trading Strategy
# RSI indicator values
rsi = 28
if rsi < 30:
print(“Strong Buy Signal”)
elif 30 <= rsi <= 70:
print(“Hold Position”)
else:
print(“Sell Position”)
Using Loops for Trade Automation
Why Use Loops in Trading?
Automates bulk order execution
Improves Freqtrade strategy efficiency
Example: Looping Through Multiple Trade Signals
# List of trade signals
signals = [“BUY BTC”, “SELL ETH”, “BUY ADA”]
for signal in signals:
print(f”Executing Trade: {signal}”)
While Loop for Real-Time Market Monitoring
Why Use While Loops?
Continuously monitors market trends
Helps execute algorithmic trading without interruptions
Example: While Loop for Price Monitoring
price = 45000
target_price = 46000
while price < target_price:
print(“Waiting for Price to Reach Target…”)
price += 1000
Go ahead—challenge yourself and solidify your learning!
Day 2: Python Operators + if-else + Loops
11/20